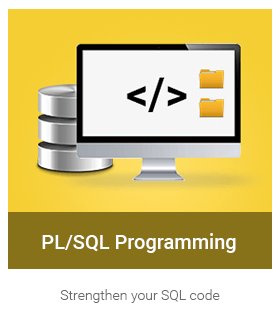
What is function ?
A Function contains a PL/SQL block to perform specific task.
The PL/SQL Function is very similar to PL/SQL Procedure. The main difference between procedure and a function is, a function must always return a value, and on the other hand a procedure may or may not return a value. Except this, all the other things of PL/SQL procedure are true for PL/SQL function too.
CREATE [OR REPLACE] FUNCTION function_name [parameters] [(parameter_name [IN | OUT | IN OUT] type [, ...])] RETURN return_datatype {IS | AS} BEGIN < function_body > END [function_name];
Here:
Function_name: specifies the name of the function.
[OR REPLACE] option allows modifying an existing function.
The optional parameter list contains name, mode and types of the parameters.
IN represents that value will be passed from outside and OUT represents that this parameter will be used to return a value outside of the procedure.
The function must contain a return statement.
RETURN clause specifies that data type you are going to return from the function.
Function body contains the executable part.
The AS keyword is used instead of the IS keyword for creating a standalone function.
Let’s see a simple example to create a function.
create or replace function add(n1 in number, n2 in number) return number is n3 number; begin n3 :=n1+n2; return n3; end; /Now write another program to call the function.
DECLARE n number(2); BEGIN n := adder(11,22); dbms_output.put_line('Addition is: ' || n); END; /PL/SQL Drop Function
Syntax for removing your created function:
DROP FUNCTION function_name;